If you’re a Javascript developer, at some point you’ve probably had a conversation like this one:
“You’re a developer? What languages do you know?”
“I mostly work with Javascript.”
“Oh, I’ve heard of Java! Cool!”
“Um…”
To the average person, these terms sound so familiar that some people don’t realize they are two separate languages. Once people find out that they are different, they often want to know which language is “better.”
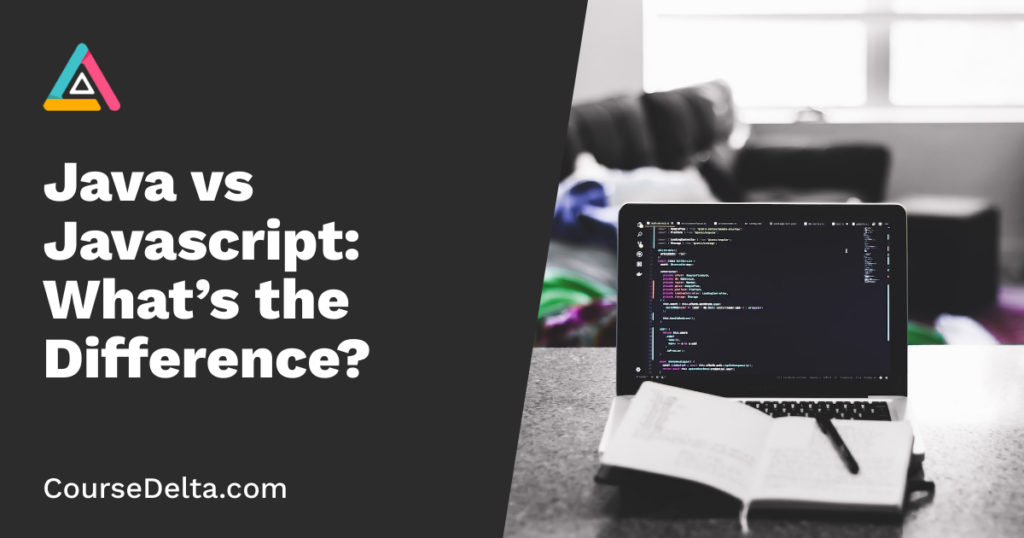
No language is necessarily “better” than another – all of them have strengths, and all of them have quirks. Different languages are used for different things. Let’s take a look at the major differences between these two languages, and when you might use one vs the other.
“Java is to JavaScript as ham is to hamster.”
Jeremy Keith
History
Java and Javascript were both developed in 1995. Java was developed at Sun as part of their core platform. Javascript, meanwhile, was developed at Netscape shortly after the release of Java.
At the time Netscape and Sun had partnered up against a common enemy: Microsoft. All three companies saw the value of the internet, and all were trying to gain a monopoly over it. Javascript, although inspired by Java, was originally called Livescript. The name was changed as a marketing ploy to ride the wave of popularity that Java was seeing.
Because of this marketing move, Javascript was misunderstood as a subset, or version of Java for a long time, when it was in fact its own language. Around the early 2000s, Douglas Crockford rediscovered Javascript in its own right, and it began to gain recognition as a serious language.
Similarities
Syntax
Java and Javascript look similar when written down. Both use curly braces to open and close code blocks, both use semicolons to end statements. They use if, while and do in similar ways. And both use return statements and there are some similar native libraries (like the Math library.)
- { }
- ;
- if () do() while()
- return
Object Oriented
Both languages make use of the object oriented programming paradigm. Object-Oriented Programming is a programming approach that breaks chunks of code into modules and defines both the structure of a module and also the methods available on it. For example, a programmer building an app where people make profiles for their pets would need a piece of code that represents a pet. The pet would be defined as an object (or class), and that object or class would have certain properties, like the pet’s name, age, photo, etc. The object would also hold any methods used for updating those properties – like changing the pet’s photo.
Differences
Classical vs Prototypal Inheritance
The main reason we organize code using Object Oriented methods is so that we can reuse pieces of code without having to rewrite them all the time. Imagine we wanted to create two versions of a User – a Man and a Woman. We could create two whole new classes – but it’s much easier to use the User class as a base.
This is where it gets tricky. Javascript classes are not like classes. They are technically not “classes” – in fact, the word class as only added to mimic Java and avoid confusion for developers who were used to using classes.
We don’t call Javascript classes “classes”: we call them “prototypes.” They’re really just functions dressed up to look like classes. There are no “public” and “private” methods or properties in Javascript prototypes, and the way that Javascript prototypes inherit from each other is different from the way Java classes inherit from each other.
Classical Inheritance (Java)
- Classes are immutable – other code cannot change them during runtime
- Classes are abstractions of objects
- One class cannot inherit from multiple parent classes
Prototypal Inheritance (Javascript)
- Prototypes can be modified by other code while the program is running
- Prototypes are objects
- A prototype can inherit from many parent objects
Javascript Inheritance
const Pet = (name, age) => { this.name = name; this.age = age; this.updateName(newName) { this.name = newName; } } |
const Dog = Object.extend({}, Pet, {voice: “bark”}); const Cat = Object.extend({}, Pet, {voice: “meow”}); |
Java Inheritance
public class Pet { String name; int age; public Pet(String name, int age) { this.name = name; this.age = age; } public String updateName(newName) { this.name = newName } } |
public class Cat extends Pet { String voice; public Cat(String voice) { this.voice = voice; } } |
Compiled vs Interpreted
Java code is compiled before it is run. The idea is that you write the code, compile it, and then you can run the compiled code anywhere. Rather than compiling all the way down to machine code, it is compiled down to JVM byte code. Machine code runs only the specific machine it was written for. JVM byte code runs on a Java virtual machine that can be installed on any system.
Javascript is not compiled before it is run – instead it is interpreted by the program running it. Instead of reading through an entire program and compiling it before execution, the interpreter compiles and executes the code line by line. Previously that interpreter could only be found in the browser, but nowadays it’s possible to interpret and run Javascript on servers too.
Typing
Java is a statically typed language – that means you define the type of a variable at the time you declare it. Variable types cannot change during program execution
String name = “Bob” int age = 17 |
Javascript is a dynamically typed language. You don’t need to declare types at the time you declare variables in your code. It also means that the type of a variable can change during execution (which can lead to some confusing bugs)
const name = “Lizzie” const age = 1 |
Concurrency
Concurrency is what programmers call many processes happening at once. For example, when loading a webpage, you might need to send a network request to a few different APIs to get the data you need. These requests all need to happen around the same time in order for the page to load properly.
Java handles concurrency with threads. Each request will have its own dedicated thread that supports it until completion.
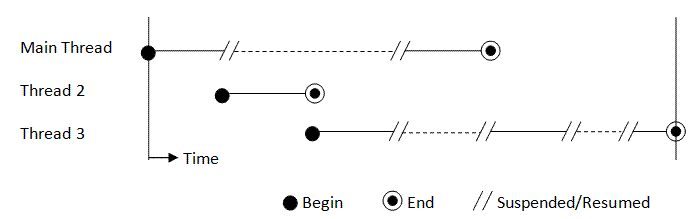
Javascript handles concurrency with an event loop. The event loop puts all requests that need to be made in a queue, and then sends one request at a time. While waiting for the first request to come back it sends the next. As each response from the server comes back, Javascript puts them into another queue and processes them in the same way.
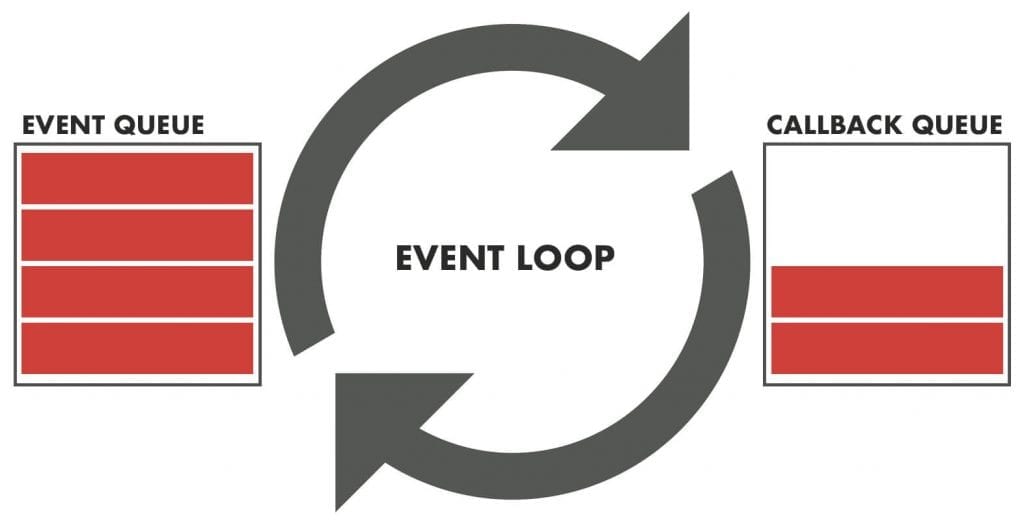
Wrapping Up
Clearly there are many differences between Java and Javascript. Each is a fully-formed language with its own idiosyncrasies and strengths. It’s a great idea for a new developer to be familiar with both. A solid understanding of both Java and Javascript will make you a more hirable engineer.